Heroes
TaleWorlds.CampaignSystem.Hero Class Reference 1.2.7
Hero.MainHero // player
Hero.OneToOneConversationHero // Hero you are talking to in the dialog
Find by StringId
Skills/Attributes/Focus/Xp
Changes
Hero.HeroDeveloper.SetInitialSkillLevel(DefaultSkills.OneHanded, 265);
Hero.MainHero.HeroDeveloper.AddAttribute(DefaultCharacterAttributes.Vigor, 1, false);
Hero.MainHero.HeroDeveloper.AddFocus(DefaultSkills.TwoHanded, 1, false);
Hero.MainHero.HeroDeveloper.ChangeSkillLevel(DefaultSkills.OneHanded, 10, true);
Hero.MainHero.HeroDeveloper.AddSkillXp(DefaultSkills.OneHanded, 100, true, true);
Hero.MainHero.AddSkillXp(DefaultSkills.Charm, MBRandom.RandomFloatRanged(10, 50)); // no learning rate applied
Get
int skillXP = hero.HeroDeveloper.GetSkillXpProgress(DefaultSkills.Athletics); // skill XP
int skillValue = hero.GetSkillValue(DefaultSkills.Roguery); // skill level
int intelligence = hero.GetAttributeValue(DefaultCharacterAttributes.Intelligence); // skill attribute
DefaultCharacterAttributes
- Vigor
- Control
- Endurance
- Cunning
- Social
- Intelligence
DefaultSkills
- OneHanded
- TwoHanded
- Polearm
- Bow
- Crossbow
- Throwing
- Riding
- Athletics
- Crafting
- Tactics
- Scouting
- Roguery
- Charm
- Leadership
- Trade
- Steward
- Medicine
- Engineering
Renown
GainRenownAction.Apply(Hero.MainHero, MBRandom.RandomFloatRanged(1f, 3f), false);
Traits
TaleWorlds.CampaignSystem.CharacterDevelopment.DefaultTraits
int Hero.GetTraitLevel(TraitObject trait)
void Hero.SetTraitLevel(TraitObject trait, int value)
void Hero.ClearTraits()
Personality traits
- Mercy - Mercy represents your general aversion to suffering and your willingness to help strangers or even enemies. Cruel tag if Mercy < 0.
- Valor - Valor represents your reputation for risking your life to win glory or wealth or advance your cause.
- Honor - Honor represents your reputation for respecting your formal commitments, like keeping your word and obeying the law. Devious tag if Honor < 0.
- Generosity - Generosity represents your loyalty to your kin and those who serve you, and your gratitude to those who have done you a favor.
- Calculating - Calculating represents your ability to control your emotions for the sake of your long-term interests.
Defined in <NPCCharacters> files, like lords.xml:
Other traits
Political:
- Egalitarian
- Oligarchic
- Authoritarian
Voice:
- PersonaCurt
- PersonaEarnest
- PersonaIronic
- PersonaSoftspoken
Profession?
- Surgery
- Siegecraft
- Blacksmith
- Fighter
- Commander
- Politician
- Manager
- Trader
- Thug
- Thief
- Gambler
- Smuggler
Skills:
- ScoutSkills
- RogueSkills
- SergeantCommandSkills
- KnightFightingSkills
- CavalryFightingSkills
- HorseArcherFightingSkills
- HopliteFightingSkills
- ArcherFIghtingSkills
- CrossbowmanStyle
- PeltastFightingSkills
- HuscarlFightingSkills
- BossFightingSkills
Equipment:
- WandererEquipment
- GentryEquipment
Body build:
- MuscularBuild
- HeavyBuild
- LightBuild
- OutOfShapeBuild
Hair:
- RomanHair
- FrankishHair
- CelticHair
- RusHair
- ArabianHair
- SteppeHair
NPC99.: Approx relationship between Lord traits and skills:
Health
bool IsHealthFull()
void Heal(int healAmount, bool addXp=false)
void MakeWounded(Hero killerHero=null, KillCharacterAction.KillCharacterActionDetail deathMarkDetail=KillCharacterAction.KillCharacterActionDetail.None)
int MaxHitPoints [get]
int HitPoints [get, set]
How to increase max health (MaxHitPoints) of the hero?
Power
float hero.Power
void hero.AddPower (float value)
Defines how powerfull a notable is (Regular [0..99], Influential [100..199], Powerful [200+])
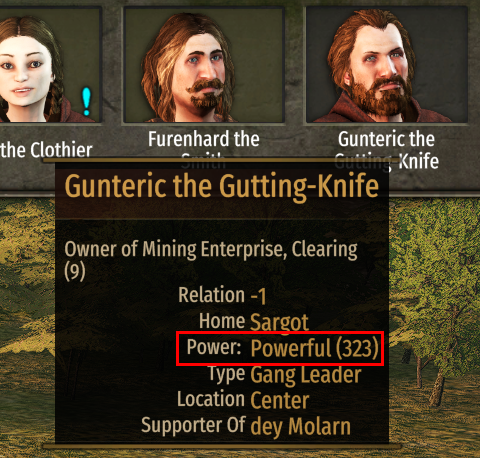
private DefaultNotablePowerModel.NotablePowerRank[] NotablePowerRanks = new DefaultNotablePowerModel.NotablePowerRank[]
{
new DefaultNotablePowerModel.NotablePowerRank(new TextObject("{=aTeuX4L0}Regular", null), 0, 0.05f),
new DefaultNotablePowerModel.NotablePowerRank(new TextObject("{=nTETQEmy}Influential", null), 100, 0.1f),
new DefaultNotablePowerModel.NotablePowerRank(new TextObject("{=UCpyo9hw}Powerful", null), 200, 0.15f)
};
A lof of stuff done in NotablePowerModel (DefaultNotablePowerModel Class), example:
int Campaign.Current.Models.NotablePowerModel.RegularNotableMaxPowerLevel
Occupation
hero.SetNewOccupation(Occupation.Special); // spawns in the keep hero.SetNewOccupation(Occupation.Wanderer); // spawns in the tavern, engine relocates to random town once a week
Occupation list
- NotAssigned
- Tavernkeeper
- Mercenary
- Lord
- GoodsTrader
- ArenaMaster
- Villager
- Soldier
- Townsfolk
- RansomBroker
- Weaponsmith
- Armorer
- HorseTrader
- TavernWench
- TavernGameHost
- Bandit
- Wanderer
- Artisan
- Merchant
- Preacher
- Headman
- GangLeader
- RuralNotable
- PrisonGuard
- Guard
- ShopWorker
- Musician
- Gangster
- Blacksmith
- BannerBearer
- CaravanGuard
- Special
Check for occupation:
- hero.IsGangLeader
- hero.IsMerchant
- hero.IsArtisan
- hero.IsLord
- hero.IsPreacher
- hero.IsWanderer
- hero.IsRuralNotable
- hero.IsUrbanNotable
- hero.IsHeadman
- hero.IsMinorFactionHero
- hero.IsNotable
Equipment
Equipment equipment = Hero.MainHero.BattleEquipment;
Equipment equipment = Hero.MainHero.CivilianEquipment;
Relations
Get relation
Use this method to get the same relation that is visible in the Encyclopedia:
This returns 'raw` relation not affected by the heroe's traits:
Set relation
CharacterRelationManager.SetHeroRelation(Hero.MainHero, otherHero, -25);
void CharacterRelationManager.SetHeroRelation(Hero hero1, Hero hero2, int value)
// use this one
ChangeRelationAction.ApplyRelationChangeBetweenHeroes(notable, clan.Leader, -20, true);
Are we at war?
Culture
Hero.Culture
Check: if (hero.Culture.StringId == "sturgia") ..
States
CharacterStates
- NotSpawned
- Active
- Fugitive
- Prisoner
- Released
- Dead
- Disabled
- Traveling
Attributes
- IsKnown - we checked him in Encyclopedia, met him
- HasMet - we actualy met him (participated in dialog)
- LastMeetingTimeWithPlayer - last time we met him
- Hero.EncyclopediaText = new TextObject("Alive and kicking!"); - Encyclopedia text
Age
hero.Age
Change:
Voice
Can be set in the lords.xml file:
Voice types:
- curt
- earnest
- ironic
- softspoken
Also depends on the culture.
Kill Character
Other ways to kill
- ApplyByOldAge // did not work in console cmd test
- ApplyByWounds
- ApplyByBattle // did not work in console cmd test
- ApplyByMurder
- ApplyInLabor
- ApplyByExecution
- ApplyByRemove // worked
- ApplyByDeathMark
- ApplyByDeathMarkForced
- ApplyByPlayerIllness
Based on how the hero was killed, Hero.DeathMark is created
Hero.DeathMark values
public enum KillCharacterActionDetail
{
None,
Murdered,
DiedInLabor,
DiedOfOldAge,
DiedInBattle,
WoundedInBattle,
Executed,
Lost
}
An obituary in the Encyclopedia depends on it (he died of natural causes/was killed/disappeared/etc):
victim.EncyclopediaText = KillCharacterAction.CreateObituary(victim, actionDetail);
Also Hero.DeathMarkKillerHero points to the killer Hero if applicable (public void AddDeathMark).
Not-wounded party heroes list
List<Hero> heroList = (from characterObject in Hero.MainHero.PartyBelongedTo.MemberRoster.GetTroopRoster()
where characterObject.Character.HeroObject != null && !characterObject.Character.HeroObject.IsWounded
select characterObject.Character.HeroObject
).ToList<Hero>();
Special Items
public List<ItemObject> SpecialItems
Conversation Tags
TaleWorlds.CampaignSystem.Conversation.Tags
List
- AlliedLordTag
- AmoralTag
- AnyNotableTypeTag
- ArtisanNotableTypeTag
- AseraiTag
- AttackingTag
- AttractedToPlayerTag
- BattanianTag
- CalculatingTag
- CautiousTag
- ChivalrousTag
- CombatantTag
- ConversationTag
- ConversationTagHelper
- CruelTag
- CurrentConversationIsFirst
- DefaultTag
- DeviousTag
- DrinkingInTavernTag
- EmpireTag
- EngagedToPlayerTag
- FirstMeetingTag
- FriendlyRelationshipTag
- GangLeaderNotableTypeTag
- GenerosityTag
- HeadmanNotableTypeTag
- HighRegisterTag
- HonorTag
- HostileRelationshipTag
- ImpoliteTag
- ImpulsiveTag
- InHomeSettlementTag
- KhuzaitTag
- LowRegisterTag
- MerchantNotableTypeTag
- MercyTag
- MetBeforeTag
- NoConflictTag
- NonCombatantTag
- NonviolentProfessionTag
- NpcIsFemaleTag
- NpcIsLiegeTag
- NpcIsMaleTag
- NpcIsNobleTag
- OldTag
- OnTheRoadTag
- OutlawSympathyTag
- PersonaCurtTag
- PersonaEarnestTag
- PersonaIronicTag
- PersonaSoftspokenTag
- PlayerBesiegingTag
- PlayerIsAffiliatedTag
- PlayerIsBrotherTag
- PlayerIsDaughterTag
- PlayerIsEnemyTag
- PlayerIsFamousTag
- PlayerIsFatherTag
- PlayerIsFemaleTag
- PlayerIsKinTag
- PlayerIsKnownButNotFamousTag
- PlayerIsLiegeTag
- PlayerIsMaleTag
- PlayerIsMotherTag
- PlayerIsNobleTag
- PlayerIsRulerTag
- PlayerIsSisterTag
- PlayerIsSonTag
- PlayerIsSpouseTag
- PreacherNotableTypeTag
- RogueSkillsTag
- RomanticallyInvolvedTag
- SexistTag
- SturgianTag
- TribalRegisterTag
- UncharitableTag
- UnderCommandTag
- UngratefulTag
- ValorTag
- VlandianTag
- VoiceGroupPersonaCurtLowerTag
- VoiceGroupPersonaCurtTribalTag
- VoiceGroupPersonaCurtUpperTag
- VoiceGroupPersonaEarnestLowerTag
- VoiceGroupPersonaEarnestTribalTag
- VoiceGroupPersonaEarnestUpperTag
- VoiceGroupPersonaIronicLowerTag
- VoiceGroupPersonaIronicTribalTag
- VoiceGroupPersonaIronicUpperTag
- VoiceGroupPersonaSoftspokenLowerTag
- VoiceGroupPersonaSoftspokenTribalTag
- VoiceGroupPersonaSoftspokenUpperTag
- WandererTag
- WaryTag
Hero XML
Add an entry into heroes.xml
<Hero id="lord_lit_1_1" faction="Faction.clan_lit_1" spouse="Hero.lord_lit_1_2" father="Hero.lit_1_0" mother="Hero.lit_1_00" alive="false" text="Encyclopedia text for the hero" />
- id - heroe's ID by which it will be referenced in other files
- faction - clan, in a format: Faction.CLAN_ID
- spouse - Hero.SPOUSE_HERO_ID
- father - Hero.FATHER_HERO_ID
- mother - Hero.MOTHER_HERO_ID
- alive - default true, set to false for dead hero
- text - Encyclopedia text
How to properly make a lord dead in XML?
alive="false" makes him dead, but in description it writes: HERO_NAME is member of ...
Lord XML
Add an entry into lords.xml
<NPCCharacter ...
<!-- Traits -->
<Traits>
<Trait id="Mercy" value="-1"/>
<Trait id="Valor" value="2"/>
<Trait id="Honor" value="0"/>
<Trait id="Generosity" value="1"/>
<Trait id="Calculating" value="2"/>
<Trait id="Egalitarian" value="-1"/>
<Trait id="Oligarchic" value="1"/>
<Trait id="Authoritarian" value="2"/>
</Traits>
Player start
SandboxCharacterCreationContent
How to change starting gear info here.
Clothes for dead heroes
Set clothes for neutral
culture. A lot of dead heroes will use this:
sandbox_equipment_sets.xml
sandboxcore_equipment_sets.xslt
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output omit-xml-declaration="yes"/>
<xsl:template match="@*|node()">
<xsl:copy>
<xsl:apply-templates select="@*|node()"/>
</xsl:copy>
</xsl:template>
<!-- dead lords gear -->
<xsl:template match="EquipmentRoster[@id='default_battle_equipment_roster_neutral']/EquipmentSet">
<EquipmentSet>
<equipment slot="Body" id="Item.tied_cloth_tunic"/>
<equipment slot="Leg" id="Item.strapped_shoes"/>
</EquipmentSet>
</xsl:template>
<xsl:template match="EquipmentRoster[@id='default_civilian_equipment_roster_neutral']/EquipmentSet">
<EquipmentSet>
<equipment slot="Body" id="Item.tied_cloth_tunic"/>
<equipment slot="Leg" id="Item.strapped_shoes"/>
</EquipmentSet>
</xsl:template>
</xsl:stylesheet>
Remaining lords will use equipment that set like this (not sure what's the difference):
spcultures.xml
default_battle_equipment_roster="EquipmentRoster.default_battle_equipment_roster_neutral"
default_civilian_equipment_roster="EquipmentRoster.default_civilian_equipment_roster_neutral">
In the code it's set as:
class Campaign
private void InitializeDefaultEquipments()
{
this.DeadBattleEquipment = Game.Current.ObjectManager.GetObject<MBEquipmentRoster>("default_battle_equipment_roster_neutral").DefaultEquipment;
this.DeadCivilianEquipment = Game.Current.ObjectManager.GetObject<MBEquipmentRoster>("default_civilian_equipment_roster_neutral").DefaultEquipment;
}